- inputThe input mesh to be modified.
C++ Type:MeshGeneratorName
Unit:(no unit assumed)
Controllable:No
Description:The input mesh to be modified.
- input_mesh_circular_boundariesNames of the circular boundaries.
C++ Type:std::vector<BoundaryName>
Unit:(no unit assumed)
Controllable:No
Description:Names of the circular boundaries.
CircularBoundaryCorrectionGenerator
This CircularBoundaryCorrectionGenerator object is designed to correct full or partial circular boundaries in a 2D mesh to preserve areas.
Overview
The CircularBoundaryCorrectionGenerator
object performs radius correction to preserve circular area considering polygonization effect for full or partial circular boundaries in a 2D mesh within the Z=0
plane.
In a 2D mesh, a "circular boundary" consists of sides that connect a series of nodes on the circle, which actually form a polygon boundary. Due to the polygonization effect, the area within a "circular boundary" in a 2D mesh is actually smaller than a real circle with the same radius. Such a discrepancy could cause issues in some simulations that involve physics that are sensitive to volume conservation.
Therefore, a corrected radius can be used to generate the "circle-like" polygon to enforce that the polygon area is the same as the original circle without polygonization. This can be achieved with either of the following approaches.
Moving Radial Nodes
The most straightforward approach for circular correction is to move the nodes on the circular boundary in their respective radial directions (see the left sub-figure of Figure 1). In that case, the azimuthal angle intervals of the boundary sides do not change. Therefore, the algorithm is relatively simple. This is also the default approach of this mesh generator.
For a polygon region used to mesh a full or partial circle, each side (with index ) corresponds to an azimuthal angle interval, , which is defined by the side and the center of the polygon:
(1)with
(2)should be for a full circle and between 0 to for a partial circle. For such a full or partial real circle, the area has the following form:
(3)To enforce that , the radii of the polygon and the circle have the following relation,
(4)Where is the correction factor used in this object to ensure volume preservation.
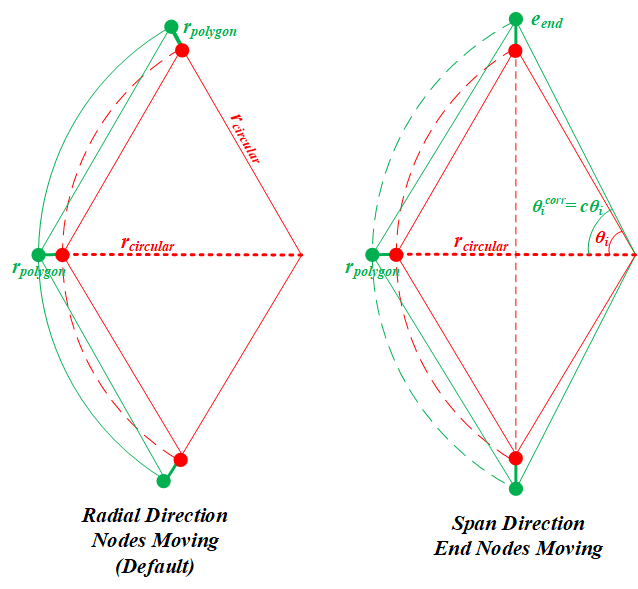
Figure 1: A schematic drawing showing the two approaches to correct the polygonization effect for a partial circular boundary
Optional additional circular boundary expansion in the span direction
Moving the radial nodes is undoubtedly the most generalized approach for a full circular boundary. However, for a partial circular boundary, moving the two end nodes in their radial directions may deform the original shape. Therefore, moving the end nodes in the span direction of the partial circular boundary (i.e., arc) provides an alternative approach (see the right subfigure of Figure 1). Moving the end nodes in the span direction inevitably changes the azimuthal angle intervals. To make this change consistent for all the boundary sides, a scaling coefficient, , is applied to every .
(5)(6)(7)Note that if the partial circular boundary happens to be a half circle (i.e., ), Eq. (7) cannot be solved as both numerator and denominator are zero. In that case the span direction is also the radial direction. Therefore, has a trivial value of unity; and the node moving should be calculated using the "radial nodes moving" approach. If the boundary if not a half circle (i.e., ), the above equation is solved by Newton-Raphson method to obtain . The displacement of the end nodes () can be calculated as follows.
(8)Users should set "move_end_nodes_in_span_direction" as true
to enable this radius correction approach for partial circular boundaries.
Usage
This object is capable of correcting multiple circular boundaries within a 2D mesh at the same time. Each circular boundary must be provided as a single boundary id/name in "input_mesh_circular_boundaries".
Moving the nodes on a circular boundary may flip the local elements. In order to reduce the probability of element flipping, a transition area is defined for each circular boundary, which is a ring area covering both inner and outer sides of the circular boundary in which the nodes will be moved based on the correction factor. The moving distance fades as the node-to-circle distance increases. The transition area size is defined using a fraction of the boundary radius, which can be customized in "transition_layer_ratios".
Example Syntax
[Mesh]
[cir1]
type = ParsedCurveGenerator
x_formula = 'r*cos(t*2*pi)'
y_formula = 'r*sin(t*2*pi)'
section_bounding_t_values = '0 1'
constant_names = 'pi r'
constant_expressions = '${fparse pi} 1.0'
nums_segments = '10'
is_closed_loop = true
[]
[cir2]
type = ParsedCurveGenerator
x_formula = 'r*cos(t*2*pi)'
y_formula = 'r*sin(t*2*pi)+0.5'
section_bounding_t_values = '0 1'
constant_names = 'pi r'
constant_expressions = '${fparse pi} 2.0'
nums_segments = '15'
is_closed_loop = true
[]
[xyd1]
type = XYDelaunayGenerator
boundary = cir1
desired_area = 0.5
refine_boundary = false
# output_boundary = '10'
output_subdomain_name = '10'
[]
[xyd2]
type = XYDelaunayGenerator
boundary = cir2
holes = xyd1
refine_holes = 'false'
desired_area = 0.5
refine_boundary = false
output_subdomain_name = '20'
hole_boundaries = '10'
[]
[ccg]
type = CircularBoundaryCorrectionGenerator
input = xyd2
input_mesh_circular_boundaries = '0 10'
custom_circular_tolerance = 1e-8
[]
[]
(moose/test/tests/meshgenerators/circular_correction_generator/radius_corr.i)Input Parameters
- custom_circular_toleranceCustom tolerances (L2 norm) used to verify whether the provided boundaries are circular (default is 1.0e-6).
C++ Type:std::vector<double>
Unit:(no unit assumed)
Controllable:No
Description:Custom tolerances (L2 norm) used to verify whether the provided boundaries are circular (default is 1.0e-6).
- move_end_nodes_in_span_directionFalseWhether to move the end nodes in the span direction of the arc or not.
Default:False
C++ Type:bool
Unit:(no unit assumed)
Controllable:No
Description:Whether to move the end nodes in the span direction of the arc or not.
- transition_layer_ratiosRatios to radii as the transition layers on both sides of the original radii (if this parameter is not provided, 0.01 will be used).
C++ Type:std::vector<double>
Unit:(no unit assumed)
Controllable:No
Description:Ratios to radii as the transition layers on both sides of the original radii (if this parameter is not provided, 0.01 will be used).
Optional Parameters
- control_tagsAdds user-defined labels for accessing object parameters via control logic.
C++ Type:std::vector<std::string>
Unit:(no unit assumed)
Controllable:No
Description:Adds user-defined labels for accessing object parameters via control logic.
- enableTrueSet the enabled status of the MooseObject.
Default:True
C++ Type:bool
Unit:(no unit assumed)
Controllable:No
Description:Set the enabled status of the MooseObject.
- save_with_nameKeep the mesh from this mesh generator in memory with the name specified
C++ Type:std::string
Unit:(no unit assumed)
Controllable:No
Description:Keep the mesh from this mesh generator in memory with the name specified
Advanced Parameters
- nemesisFalseWhether or not to output the mesh file in the nemesisformat (only if output = true)
Default:False
C++ Type:bool
Unit:(no unit assumed)
Controllable:No
Description:Whether or not to output the mesh file in the nemesisformat (only if output = true)
- outputFalseWhether or not to output the mesh file after generating the mesh
Default:False
C++ Type:bool
Unit:(no unit assumed)
Controllable:No
Description:Whether or not to output the mesh file after generating the mesh
- show_infoFalseWhether or not to show mesh info after generating the mesh (bounding box, element types, sidesets, nodesets, subdomains, etc)
Default:False
C++ Type:bool
Unit:(no unit assumed)
Controllable:No
Description:Whether or not to show mesh info after generating the mesh (bounding box, element types, sidesets, nodesets, subdomains, etc)