- cornersThe x,y,z positions of the nodes
C++ Type:std::vector<libMesh::Point>
Controllable:No
Description:The x,y,z positions of the nodes
TransfiniteMeshGenerator
The TransfiniteMeshGenerator
produces two-dimensional meshes from a set of 4 corner vertices by interpolating the edges into the inner domain. The resulting mesh consists of QUAD4 elements of aspect ratio and skewness determined by the points on the boundary edges.
Creates a QUAD4 mesh given a set of corner vertices and edge types. The edge type can be either LINE, CIRCARC, DISCRETE or PARSED, with LINE as the default option. For the non-default options the user needs to specify additional parameters via the edge_parameter option as follows: for CIRCARC the deviation of the midpoint from an arccircle, for DISCRETE a set of points, or a paramterization via the PARSED option. Opposite edges may have different distributions s long as the number of points is identical. Along opposite edges a different point distribution can be prescribed via the options bias_x or bias_y for opposing edges.
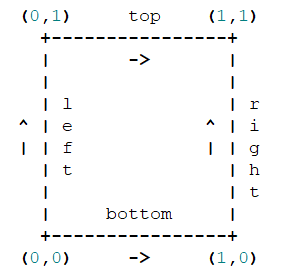
Fig. 1: Convention for the corner vertices order.
Overview
Given a set of 4 corners the TransfiniteMeshGenerator constructs by default the straight edges that connect the vertices and fills up the interior with straight lines. The test, quadrilater_generator.i, illustrates the use of the TransfiniteMeshGenerator
object to construct general quadrilaterals as in Fig. 2. By default the distribution of points along edges is considered equidistant, unless specified otherwise by the user using the parameters bias_x
or bias_y
. The core of the algorithm interpolates/fills up the inner domain for any edge type, curved, parametrized, discrete. The current implementation considers all these cases with special attention given to arcs of a circle which are widely encountered in scientific computing applications.
The convention for the corners numbering should follow Fig. 1, prescribed in the order (0,0) - (0,1) - (1,1) - (1,0). The direction along edges, important if the user provides them using the DISCRETE option, should follow the direction of the arrows as in Fig. 1.
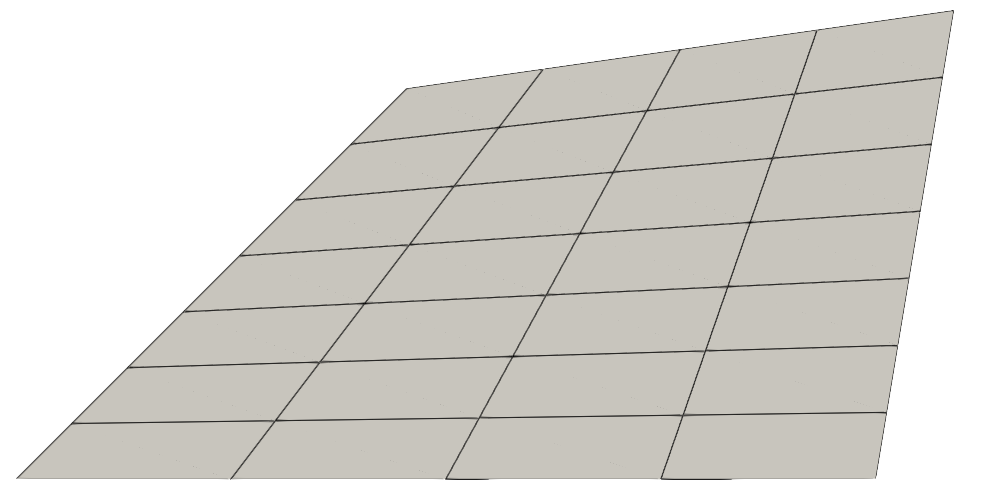
Fig. 2: A quadrilateral with one edge on an arc circle.
This generator allows any type of curvilinear edges. In Fig. 3 we show a mesh where the top edge has an inward arc circle. However, this algorithm generates a mesh for any parametrized edge, or given set of points on an edge. For arcs of a circle, since they are widely encountered in applications, we customized an approach that requires a user to specify only the deviation from a straight line in the middle of an edge.
The default distribution of points on an edge is equidistant and nothing explicit needs to be specified. However, for meshes that need to capture more scales in different regions we have two additional options that can be prescribed. The distribution of points on an edge can be either provided as a discrete set of points, using the edge type DISCRETE
, or as a bias along the edge. The only requirement is that opposite edges have the same number of points.
Example
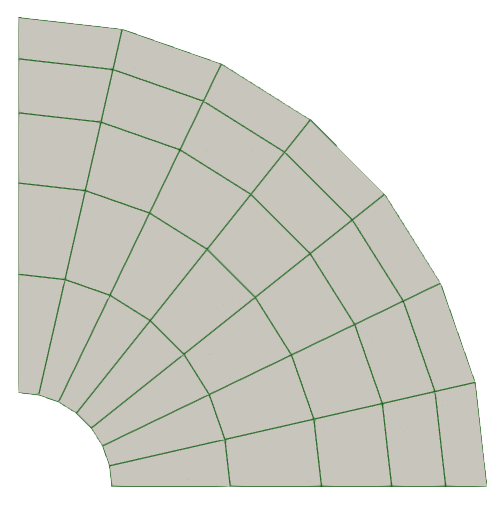
Fig. 3: Annulus section generated using CIRCARC
edges.
This mesh generator considers 4 types of edges, each of which should have a type defined in "edge"_type
, where "edge" is either left
, right
, top
or bottom
. For some edge types, an edge parameter, similarly named "edge"_parameter
, is necessary to define the shape of the edge.
Admissible types are:
- LINE
- constructs a line between the already-provided corner vertices. A quadrilateral can be obtained only with line sides as in Fig. 2.
- CIRCARC
- constructs an arc of a circle, based on a required edge parameter. This parameter can be the distance at the middle of an edge between a straight line and the arc of circle desired, as in the test arccircle_generator.i. The edge is then generated by computing the middle point on the edge. The deviation from a straight line is set by convention to be positive if the arc circle is outward, or negative if inward, in this fashion the user can specify different orientations, as in Fig. 4. The midpoint could instead be obtained from a QUAD8 element as provided by an external mesh generator and inserted as input by the user in the same edge parameter, as in the test arccircle_midpoint_generator.i. Different point distributions along arc circles can be prescribed as for the other edge types and propagate via the polar coordinates parametrization.
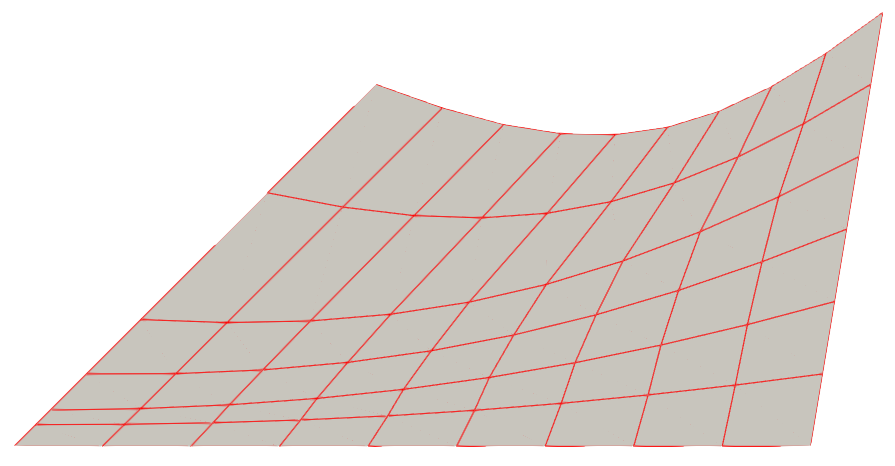
Fig. 4: A domain with edge types CIRCARC
and DISCRETE
.
[Mesh<<<{"href": "../../syntax/Mesh/index.html"}>>>]
[transf]
type = TransfiniteMeshGenerator<<<{"description": "Creates a QUAD4 mesh given a set of corner vertices and edge types. The edge type can be either LINE, CIRCARC, DISCRETE or PARSED, with LINE as the default option. For the non-default options the user needs to specify additional parameters via the edge_parameter option as follows: for CIRCARC the deviation of the midpoint from an arccircle, for DISCRETE a set of points, or a paramterization via the PARSED option. Opposite edges may have different distributions s long as the number of points is identical. Along opposite edges a different point distribution can be prescribed via the options bias_x or bias_y for opposing edges.", "href": "TransfiniteMeshGenerator.html"}>>>
corners<<<{"description": "The x,y,z positions of the nodes"}>>> = '-1.0 -1.0 0.0
10.0 -1.0 0.0
11.0 5.0 0
4.0 4.0 0'
nx<<<{"description": "Number of nodes on horizontal edges, including corners"}>>> = 10
ny<<<{"description": "Number of Nodes on vertical edges, including corners"}>>> = 7
left_type<<<{"description": "type of the left (x) boundary"}>>> = DISCRETE
right_type<<<{"description": "type of the right (x) boundary"}>>> = LINE
top_type<<<{"description": "type of the top (y) boundary"}>>> = CIRCARC
bottom_type<<<{"description": "type of the bottom (y) boundary"}>>> = LINE
left_parameter<<<{"description": "Left side support parameter"}>>> = '-1.0 -1.0 0
-0.7 -0.7 0.0
-0.5 -0.5 0.0
0.0 0.0 0.0
0.75 0.75 0.0
2.5 2.5 0
4.0 4.0 0.0'
top_parameter<<<{"description": "Top side support parameter"}>>> = '-1.17'
[]
[]
(moose/test/tests/meshgenerators/transfinite_generator/discrete_generator.i) - DISCRETE
- constructs a line along a set of points, provided by the user in a required edge parameter. Coordinates for each point are separated by whitespace, and points are separated by newlines. Points are connected in the order represented by arrows in Fig. 1. A test is available in discrete_generator.i and illustrated in Fig. 4. This option is the only one that does not support a different point redistribution via the options bias_x
and bias_y
; these will have no effect, since it is assumed the user desires their edge points exactly as specified.
- PARSED
- constructs a curvilinear edge, as provided by a parametric function specified in the corresponding edge parameter. In this parameter, the function to be parsed should be a map from the unit interval r=[0,1]
, with the output x
and y
components separated by a semicolon. The test parsed_generator.i illustrates this by constructing a stenotic pipe mesh using parsed top and bottom edges.
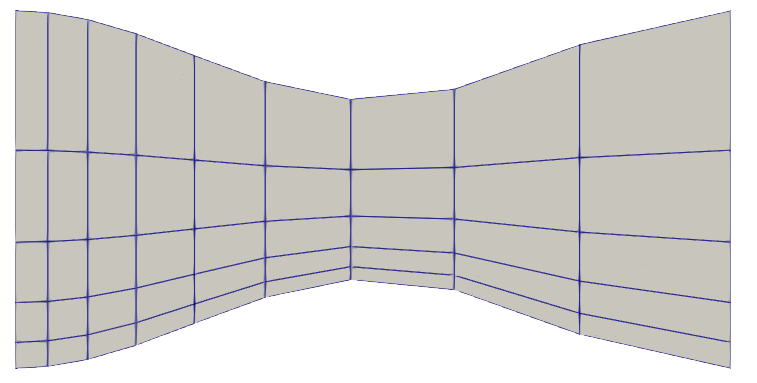
Fig. 5: A stenotic pipe generated using parametrized edges using PARSED
and different point distributions (bias_x
, bias_y
).
[Mesh<<<{"href": "../../syntax/Mesh/index.html"}>>>]
[transf]
type = TransfiniteMeshGenerator<<<{"description": "Creates a QUAD4 mesh given a set of corner vertices and edge types. The edge type can be either LINE, CIRCARC, DISCRETE or PARSED, with LINE as the default option. For the non-default options the user needs to specify additional parameters via the edge_parameter option as follows: for CIRCARC the deviation of the midpoint from an arccircle, for DISCRETE a set of points, or a paramterization via the PARSED option. Opposite edges may have different distributions s long as the number of points is identical. Along opposite edges a different point distribution can be prescribed via the options bias_x or bias_y for opposing edges.", "href": "TransfiniteMeshGenerator.html"}>>>
corners<<<{"description": "The x,y,z positions of the nodes"}>>> = '-1.0 -0.5 0.0
1.0 -0.5 0.0
1.0 0.5 0.0
-1.0 0.5 0.0'
nx<<<{"description": "Number of nodes on horizontal edges, including corners"}>>> = 10
ny<<<{"description": "Number of Nodes on vertical edges, including corners"}>>> = 6
bottom_type<<<{"description": "type of the bottom (y) boundary"}>>> = PARSED
top_type<<<{"description": "type of the top (y) boundary"}>>> = PARSED
right_type<<<{"description": "type of the right (x) boundary"}>>> = LINE
left_type<<<{"description": "type of the left (x) boundary"}>>> = LINE
top_parameter<<<{"description": "Top side support parameter"}>>> = '2*r-1 ; 3/8 - cos(pi*(2*r-1))/8'
bottom_parameter<<<{"description": "Bottom side support parameter"}>>> = '2*r-1 ; cos(pi*(2*r-1))/8 - 3/8'
bias_x<<<{"description": "The amount by which to grow (or shrink) the cells in the x-direction."}>>> = 1.21
bias_y<<<{"description": "The amount by which to grow (or shrink) the cells in the y-direction."}>>> = 1.52
[]
[]
(moose/test/tests/meshgenerators/transfinite_generator/parsed_biased_generator.i)Since opposite sides need to have the same number of points, we consider top-bottom edges to have nx
points, and left-right edges ny
points. By default they are taken to be equidistant, unless the user specifies a distancing via the parameters bias_x
or bias_y
. Different point distributions can propagate on curvilinear edges, generating domains as in Fig. 5. Note that the bias along an edge is implemented as propagated by the parametrization, so for a parametrized edge the bias is constructed first for the parameter and propagated as the parametrized edge is generated.
Input Parameters
- epsilon0Fuzzy comparison tolerance
Default:0
C++ Type:double
Unit:(no unit assumed)
Controllable:No
Description:Fuzzy comparison tolerance
Optional Parameters
- bias_x1The amount by which to grow (or shrink) the cells in the x-direction.
Default:1
C++ Type:double
Unit:(no unit assumed)
Controllable:No
Description:The amount by which to grow (or shrink) the cells in the x-direction.
- bias_y1The amount by which to grow (or shrink) the cells in the y-direction.
Default:1
C++ Type:double
Unit:(no unit assumed)
Controllable:No
Description:The amount by which to grow (or shrink) the cells in the y-direction.
- nxNumber of nodes on horizontal edges, including corners
C++ Type:unsigned int
Controllable:No
Description:Number of nodes on horizontal edges, including corners
- nyNumber of Nodes on vertical edges, including corners
C++ Type:unsigned int
Controllable:No
Description:Number of Nodes on vertical edges, including corners
Number And Distribution Of Points Parameters
- bottom_parameterBottom side support parameter
C++ Type:std::string
Controllable:No
Description:Bottom side support parameter
- left_parameterLeft side support parameter
C++ Type:std::string
Controllable:No
Description:Left side support parameter
- right_parameterRight side support parameter
C++ Type:std::string
Controllable:No
Description:Right side support parameter
- top_parameterTop side support parameter
C++ Type:std::string
Controllable:No
Description:Top side support parameter
Edge Parameters
- bottom_typetype of the bottom (y) boundary
C++ Type:MooseEnum
Controllable:No
Description:type of the bottom (y) boundary
- left_typetype of the left (x) boundary
C++ Type:MooseEnum
Controllable:No
Description:type of the left (x) boundary
- right_typetype of the right (x) boundary
C++ Type:MooseEnum
Controllable:No
Description:type of the right (x) boundary
- top_typetype of the top (y) boundary
C++ Type:MooseEnum
Controllable:No
Description:type of the top (y) boundary
Edge Type Parameters
- disable_fpoptimizerFalseDisable the function parser algebraic optimizer
Default:False
C++ Type:bool
Controllable:No
Description:Disable the function parser algebraic optimizer
- enable_ad_cacheTrueEnable caching of function derivatives for faster startup time
Default:True
C++ Type:bool
Controllable:No
Description:Enable caching of function derivatives for faster startup time
- enable_auto_optimizeTrueEnable automatic immediate optimization of derivatives
Default:True
C++ Type:bool
Controllable:No
Description:Enable automatic immediate optimization of derivatives
- enable_jitTrueEnable just-in-time compilation of function expressions for faster evaluation
Default:True
C++ Type:bool
Controllable:No
Description:Enable just-in-time compilation of function expressions for faster evaluation
- evalerror_behaviornanWhat to do if evaluation error occurs. Options are to pass a nan, pass a nan with a warning, throw a error, or throw an exception
Default:nan
C++ Type:MooseEnum
Controllable:No
Description:What to do if evaluation error occurs. Options are to pass a nan, pass a nan with a warning, throw a error, or throw an exception
Parsed Expression Advanced Parameters
- enableTrueSet the enabled status of the MooseObject.
Default:True
C++ Type:bool
Controllable:No
Description:Set the enabled status of the MooseObject.
- save_with_nameKeep the mesh from this mesh generator in memory with the name specified
C++ Type:std::string
Controllable:No
Description:Keep the mesh from this mesh generator in memory with the name specified
Advanced Parameters
- nemesisFalseWhether or not to output the mesh file in the nemesisformat (only if output = true)
Default:False
C++ Type:bool
Controllable:No
Description:Whether or not to output the mesh file in the nemesisformat (only if output = true)
- outputFalseWhether or not to output the mesh file after generating the mesh
Default:False
C++ Type:bool
Controllable:No
Description:Whether or not to output the mesh file after generating the mesh
- show_infoFalseWhether or not to show mesh info after generating the mesh (bounding box, element types, sidesets, nodesets, subdomains, etc)
Default:False
C++ Type:bool
Controllable:No
Description:Whether or not to show mesh info after generating the mesh (bounding box, element types, sidesets, nodesets, subdomains, etc)